
In most cases when building a website with WordPress, the theme you activate will by default display a front page that lists your recent posts unless you choose to display a static page from the admin options under Settings > Reading. But what if you wanted to display a different range of content that was neither a static page nor your latest posts? Fortunately this is possible using WordPress Multiple Loops.
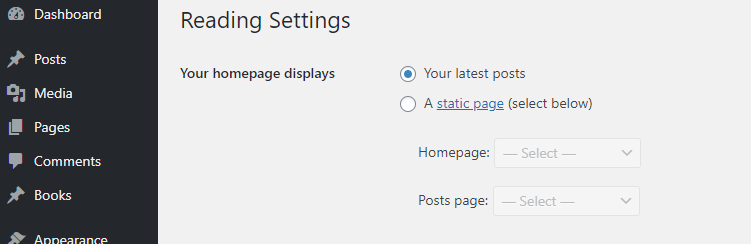
Let’s see how this can be accomplished!
Use Multiple Loops on your WordPress Homepage
Before we kick off it’s worth noting that you should carry out these modifications to your own website using a child theme as we’ll be editing your theme’s template files. If you don’t do this, you’ll find you’ll lose any modifications you make the next time you update your theme. You can find out more about child themes here.
No matter the theme you are using, the corresponding PHP file for the homepage will include a standard loop that displays some Posts (in most cases this will be recently published posts). In the Twenty Twenty theme for example, you’ll find the code that controls this inside the index.php
file around line 79.
if ( have_posts() ) {
$i = 0;
while ( have_posts() ) {
$i++;
if ( $i > 1 ) {
echo '<hr class="post-separator styled-separator is-style-wide section-inner" aria-hidden="true" />';
}
the_post();
get_template_part( 'template-parts/content', get_post_type() );
}
}
In a standard loop like this, two important global variables are used:
- The
$wp_query
which is aWP_Query
class object and holds a WP database query result - The
$post
which is the currentWP_Post
class object.
In the piece of code above, we first check if there are any posts to show with the have_posts()
global function and, if so, iterate through these posts inside a while loop. The the_post()
global function is the one that iterates through posts telling WordPress to go to the next post.
If you want to see these functions and classes in details, you can find them in the wp-includes/class-wp-query.php
file.
For the purposes of our example we activated the Twenty Twenty theme and then created 6 Posts under a category called “Category 1” and 5 Service Posts under a category named “Services”.
To keep things easy we will keep the loop code as simple as possible by displaying only a list of the Posts titles.
if ( have_posts() ) {
while ( have_posts() ) {
the_post();
the_title( '<h3 class="entry-title">', '</h3>' );
}
}
This means in our homepage we will see something like this:
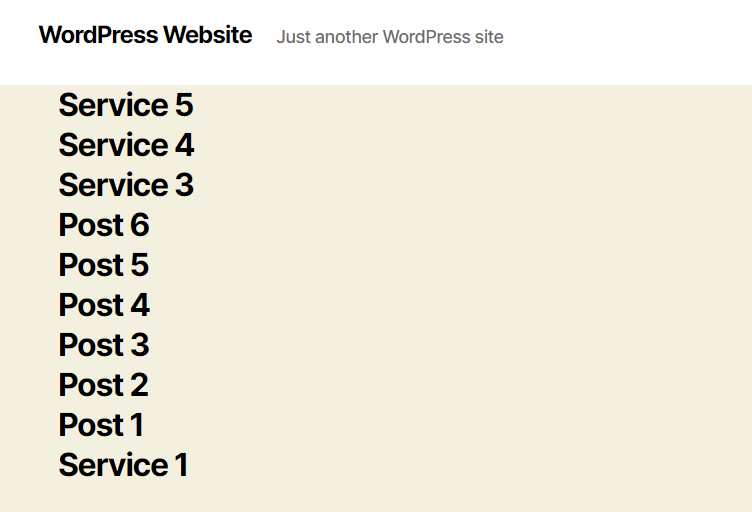
Getting Started Customizing our Homepage Output
As we saw from the screenshot above, if we visit our ‘front page’ we’ll see the 10 most recent posts regardless of the categories that have been assigned to them.
We’ll now proceed with creating our own custom homepage template where we will include customized output.
To do this, create a file called custom-homepage.php
and save it in your theme’s folder. Add the following code to this page:
<?php
/*
* Template Name: My custom homepage template
*/
get_header();
if ( have_posts() ) {
while ( have_posts() ) {
the_post();
the_title( '<h4 class="example"></h4>' );
}
}
get_footer();
Then, head to your WordPress Admin and create a page called ‘My Homepage’ and select ‘My custom homepage template’ from the Template dropdown menu as it’s template.
Then to make this the default Homepage you’ll need to select this in the Settings menu (Settings > Reading > A Static Page).
At this point, if you take a look at your Homepage you should see only the page title when viewed from the front end of your website.
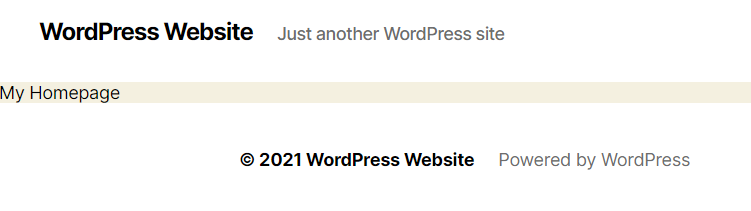
Now we’ve got this setup, let’s add some queries to our ‘My custom homepage template’ template.
Adding Custom Queries
Our goal in this example will be to display some of our latest posts from all categories. Below this we will display posts from a custom post type called ‘books’. If you don’t know how to setup Custom Post Types then checkout are article ‘Create WordPress Custom Post Types Manually‘. Including a Custom Post Type is of course, entirely optional. If you don’t want to do this then just skip the steps where we refer to this Post type.
Try our Award-Winning WordPress Hosting today!
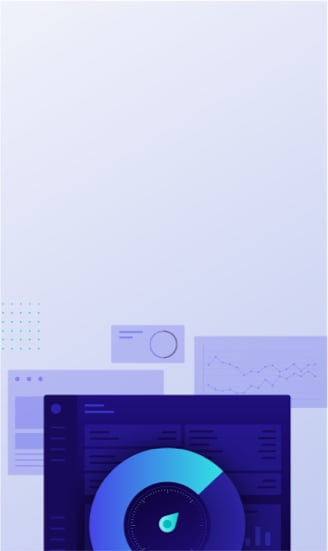
Let’s begin by replacing the current loop (found in index.php
at around line 79) with this one:
$args = array(
'posts_per_page' => 8,
'orderby', 'date',
'order', 'DESC'
);
$query = new WP_Query( $args );
while ( $query->have_posts() ) :
$query->the_post();
the_title( '<h4 class="example"></h4>' );
endwhile;
This loop will mean your Homepage will now display your 8 most recent posts (as shown below):
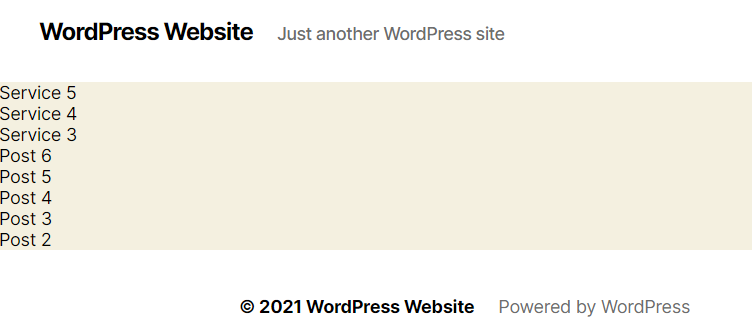
Then, if you want to include the custom post type ‘books’ (or any other custom post type for that matter) we will add a second loop for the book posts right under the first loop like this:
echo '<h4>Books</h4>';
$args = array(
'post_type' => 'book',
'posts_per_page' => 8
);
$query = new WP_Query( $args );
while ($query->have_posts()) :
$query->the_post();
the_title( '<h4 class="example"></h4>' );
endwhile;
Head to the front end of your website and refresh to the page to confirm everything is working as expected.
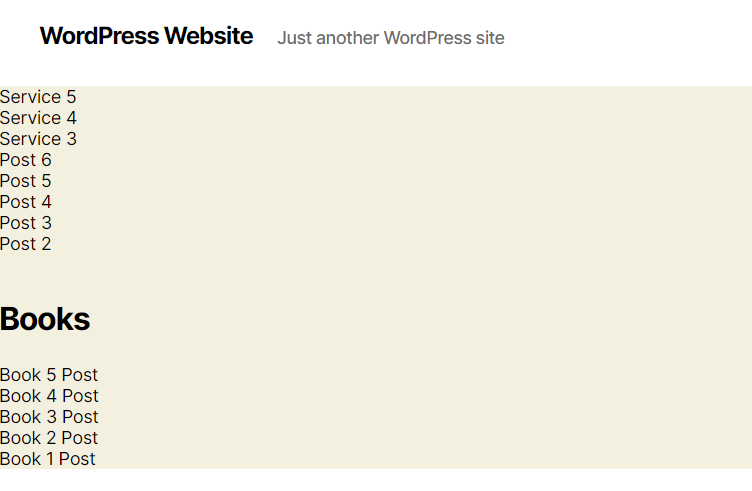
Break a Custom Query in Parts
It can be useful to break a custom query into parts. A reason for doing this would be if you wanted to ‘interrupt’ the first query by showing posts from another category or post type before then resuming the initial loop. This is useful if for example, you want to show the latest posts from a category and then show other content before finally concluding with more ‘latest posts’ to finish off the page.
Let’s jump into this. To begin, delete the contents of the custom-homepage.php
file we used before and add in the code below.
<?php
/*
* Template Name: My custom homepage template
*/
get_header();
////////////
// Services Posts loop
echo '<h4>Services</h4>';
$args = array(
'posts_per_page' => 3,
'category_name'=> 'services'
);
$services = new WP_Query( $args );
while ( $services->have_posts() ) :
$services->the_post();
the_title( '<h4 class="example"></h4>' );
endwhile;
// Books Posts in between
echo '<h4>Books</h4>';
$args = array(
'post_type' => 'book',
);
$books = new WP_Query( $args );
while ( $books->have_posts() ) :
$books->the_post();
the_title( '<h4 class="example"></h4>' );
endwhile;
// Resume Services Posts loop
echo '<h4>More Services</h4>';
$args = array(
'offset' => '3',
'posts_per_page' => 3,
'category_name'=> 'services'
);
$services = new WP_Query( $args );
while ( $services->have_posts() ) :
$services->the_post();
the_title( '<h4 class="example"></h4>' );
endwhile;
////////////
get_footer();
In the first loop we retrieve 3 posts from the Services category. In order to resume the Services category posts in the third loop whilst avoiding duplicates, we define an offset => 3
. This would mean we’d see the following:
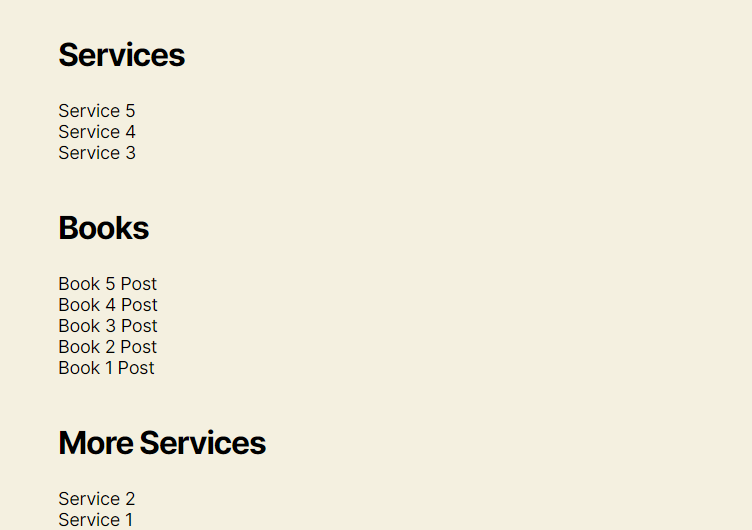
Of course you could alter the offset value to any number you wish.
Add Custom Posts to the Latest Posts Query
What if we want to include the Book Posts in the homepage latest posts? The default loop does not by default include custom posts. Here is how we can fix this.
For this section we will set the reading settings for the homepage to ‘Your latest posts’.
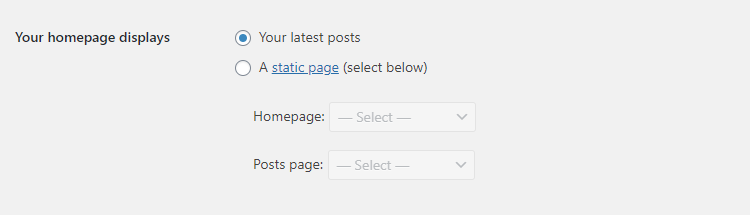
The route to follow here is the use of the pre_get_posts
hook. This hook is introduced in the wp-includes/class-wp-query.php
file and is used to customize the $query
object and main WordPress Loop as you see fit. It executes after the query variable object is created, but before the actual query is run.
Open your active theme’s functions.php
file and insert the following piece of code at the end:
function homepage_add_cpt( $query ) {
if ( $query->is_main_query() && is_home() ) {
$query->set( 'post_type', array( 'post', 'book' ) );
}
}
add_action( 'pre_get_posts', 'homepage_add_cpt' );
What this code does in the if
condition is to make sure the modifications are applied in the homepage only while the main query is running.
If you now visit your homepage you will notice that in the latest posts, the custom ‘book’ posts are also included.
Conclusion
Pretty much without exception, whatever you need to achieve in terms of content for your WordPress homepage is possible. As we’ve shown here, using WordPress Multiple Loops is a powerful way of customizing the output that appears on your Homepage. Our approach here is just one of many. WordPress offers incredible levels of control over the output that appears on your site. Experiment with the tools they provide to achieve what you want!
Start Your 14 Day Free Trial
Try our award winning WordPress Hosting!
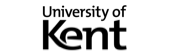
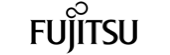
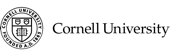
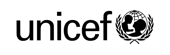
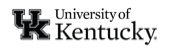