
If you are a WordPress developer it is likely that you write your code in a procedural way. You set some simple ordered steps and follow them to achieve the desired output and solve a problem. A simple example is a WordPress loop like the one shown below:
<?php
if ( have_posts() ) {
while ( have_posts() ) {
the_post();
//
// Post Content here
//
} // end while
} // end if
?>
In a loop, you first check whether you have posts that correspond to the running query, and if so, you start looping through the posts with the while loop. The same goes for every custom function you will insert in the functions.php
file.
In this article, we will help you get familiar with the OOP concept and mindset before proceeding with our plugin development.
We’re going to transition away from procedural programming and look at a style of programming called Object-Oriented Programming (OOP). We will examine how this is different from what you’ve used so far as a programming style and take a look at some of its advantages. But most importantly, we will explain how object-oriented concepts work in a WordPress environment and the relation between them.
What we would like to establish before we get further into this is that it is important that you learn about the role of object-oriented programming and when it’s suitable to be used. Learning how to build an object-oriented plugin or theme may be suitable for your project, but it’s important you understand when this is the case. Hopefully, this article will help you understand when and why a certain project may be suitable to be written in object-oriented code.
The Problem
WordPress itself has already pushed you into thinking procedurally. And, though WordPress uses PHP objects all the time, it doesn’t follow that WordPress itself is Object-Oriented. This is a common misinterpretation of what OOP is about and the reason many people believe WordPress is Object-Oriented although it’s not.
It’s important to clarify that we’re not saying you should refrain from using procedural programming, but it is true that there are limitations to this coding style. For example, when customizing a theme or plugin, it is likely that you will achieve the desired HTML output by writing code in a procedural way. But what about scalability and future maintenance?
When building a custom plugin, it is very important that you take stock and assess how scalable it will be. For example, you will probably want to be able to grow your plugin by adding further features as the plugin matures. It’s at this point where you will start having difficulties organizing your code when you code procedurally.
But even if you’re not coding plugins, with procedural programming, you always take the risk of reaching a point where you’re not sure how everything fits together anymore. As a result, every small change you make may have unintended consequences.
Object-oriented programming is used to solve more complicated problems. It may share the same goal as procedural programming, but in many cases, it offers a superior way of working. It allows you to create bold solutions to a problem that are reusable, organized, and secure.
How Object-Oriented Programming Solves Problems
Object-oriented programming has two important concepts: Classes and Objects.
Classes are essentially templates used to make objects. Each object has its own methods and set of properties and the value of those properties may vary.
So before moving forward, it is vital to make sure we understand what an Object is. Let’s break down a simple sentence as an example:
‘I eat green apples’.
Apple is the Object, green is the color of the Object (an Object property), and the verb ‘eat’ is the method (function).
A Class defines the properties and behavior of all objects that use it. Solving problems with OOP is achieved by combining these programming ‘bricks’ the correct way.
Before diving in, an important first step is to take some time to design your proposed solution. In essence, what you need to do is:
- Define the problem and what the plugin should do to solve it
- Describe the classes and their relationships as well as the interaction between the objects
- Turn everything into code
- Review and test the project
While you are at this step, keep in mind that you’ll be lucky if you get it perfect at the first attempt! You will need to start over until you get the right concept that will solve the problem. Consider it as part of a creative process that will offer you a set of tools you can later use as a team with no conflicts.
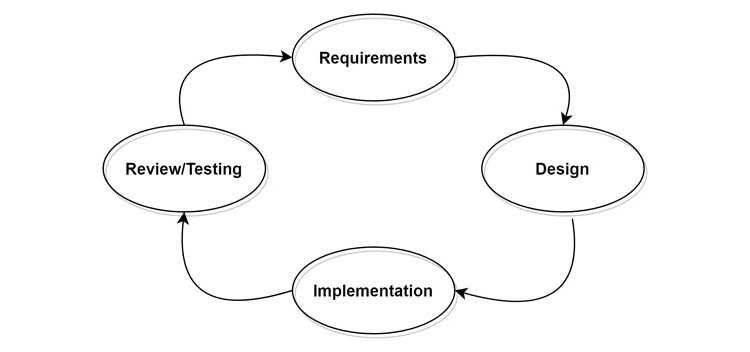
In a next article related to OOP we will demonstrate a simple example that will bring all the above theory to life.
The Features of Object-Oriented Programming
Before we get into the features of Object-Orientated programming let’s see if we can further clarify how it works with a simple metaphor.
Let’s say we have a boiler that we use to boil some water. When we use the machine, we do it in a certain way, the only way the manufacturer allows us. We cannot override the way the boiler runs when we press the ‘On’ button and, to be honest, neither would we want or need to. That gives us two very important advantages.
First of all, the limited functionality (i.e. to boil some water) means the system is both more robust and more secure. The limited feature set and the provision of just an ‘On/Off’ button mean we, as the user, can’t get anywhere near the mechanism of the boiler. By default, this means there is less to go wrong and the system is more robust as a whole. The simplicity of the operation also makes it much more user friendly with pretty much anyone able to use it.
Try our Award-Winning WordPress Hosting today!
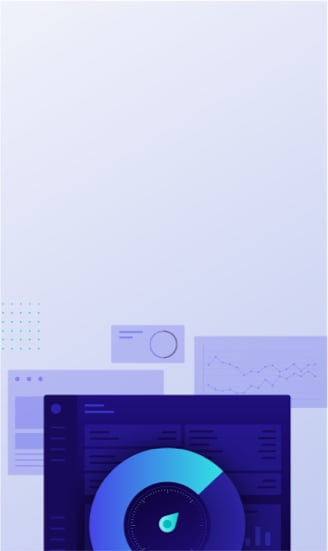
Keeping this in mind let’s see how this relates to Object-Oriented programming. In theory, OOP has three valuable characteristics: Encapsulation, Inheritance and Polymorphism.
What Encapsulation does is to group the data and behaviour inside a single entity. Inside the Class, you can define how it will be used (boiler button) and control the visibility by using the Public, Protected or Private labelling on Classes. This is what also makes it a very secure system because it gives you the ability to control who has access. This also means it’s easier to maintain and also use.
Inheritance is about helping you reuse code between your classes. One such example is when a Class extends another Class. You might have used this when extending the Walker Class to change the HTML output of a tree-like data like a menu or comments structure. Understanding the relationship between your classes will enable you create reusable object-oriented code.
Finally Polymorphism is what makes all those relationships work together, what defines what your Classes have in common, how to reuse these common elements and whether they are related to anything else.
Conclusion
Without some concrete examples it’s likely that all of the above is still a little confusing. It’s none-the-less important however to spell out the theory behind Object-Oriented programming and in future articles we’ll move this forward with some useful examples so you can see how to use this exciting programming method in the real world.
Our goal is that when you conclude reading our series of articles on OOP, you’ll be confident to use this programming method in a wide variety of situations. In the meantime you might want to carry on your reading about OOP on Wikipedia.
Next, in Part 2 in our Objected Oriented Programming Series, we explain this different approach and mindset by using a real world scenario.
See Also
- Summary of the series
- Part 3 – WordPress and Object Oriented Programming: A WordPress Example – Defining the Scope
- Part 4 – WordPress and Object Oriented Programming: A WordPress Example – Design
- Part 5 – WordPress and Object Oriented Programming: A WordPress Example – Implementation: The Administration Menu
- Part 6 – WordPress and Object Oriented Programming: A WordPress Example – Implementation: Registering the Sections
- Part 7 – WordPress and Object Oriented Programming: A WordPress Example – Implementation: Managing WordPress Hooks
- Part 8 – WordPress and Object Oriented Programming: A WordPress Example – Implementation: Options
Start Your 14 Day Free Trial
Try our award winning WordPress Hosting!
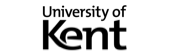
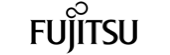
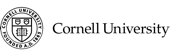
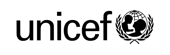
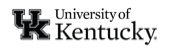